Today I'm gonna write about an advanced use case in cross-platform mobile development. Yeah! New Year New Me!
Let's say you want your RN app to use the android native calendar UI and store an event in the users Google Calendar. How ? There's no actual way in RN, as of right now! However there's hope.
A native module is a set of javascript functions that are implemented natively for each platform (in our case is iOS and Android). It is used in cases where native capabilities are needed, that react native doesn't have a corresponding module yet, or when the native performance is better.
Let's dive in.
1. Create your RN app as usual. We do need the android folder so eject if you have to.
2. Open the src folder in Android Studio or VS code - we need to edit some Java source files.
3. Under src/main/java directory create a file for your custom module. I named mine CalendarModule.java since it's about calendar.
Basically, we need to create a bridge(api) between the native code (java) and react (js)
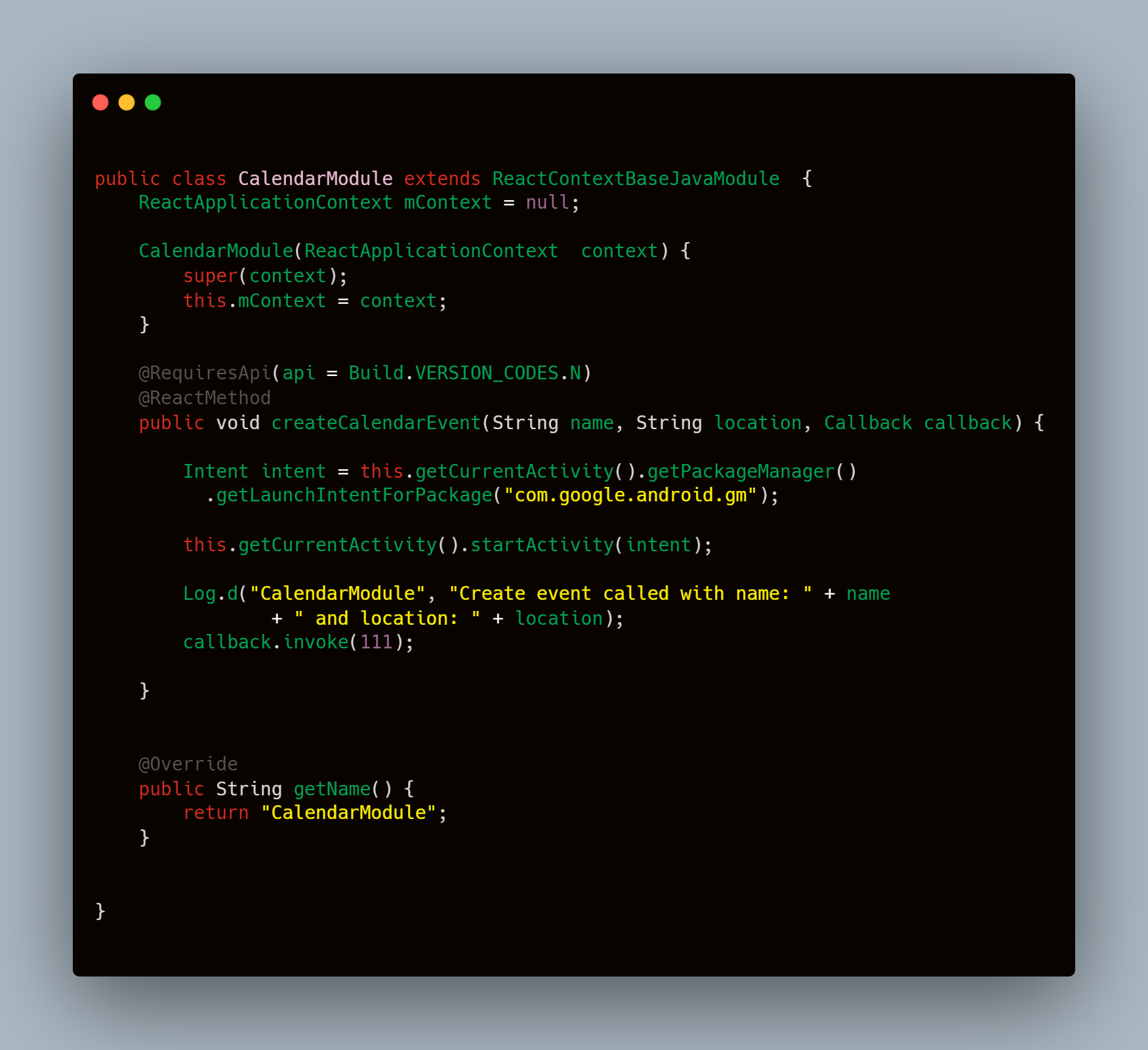
What you must understand here:
The class must extend ReactContextBaseJavaModule which allows the class to be recognized as a bridged module
Annotation @ReactMethod will be followed by one of Native Android API's. In this case createCalendarEvent(...). This method will need a Name for the event, a location and optionally a Callback. This is the code that shows the calendar UI and stores the event in users Google Calendar.
Implementing the getName() method is mandatory - that's the name we will use to make the call from RN
Last thing - open AppPackage and list the new created module like below:
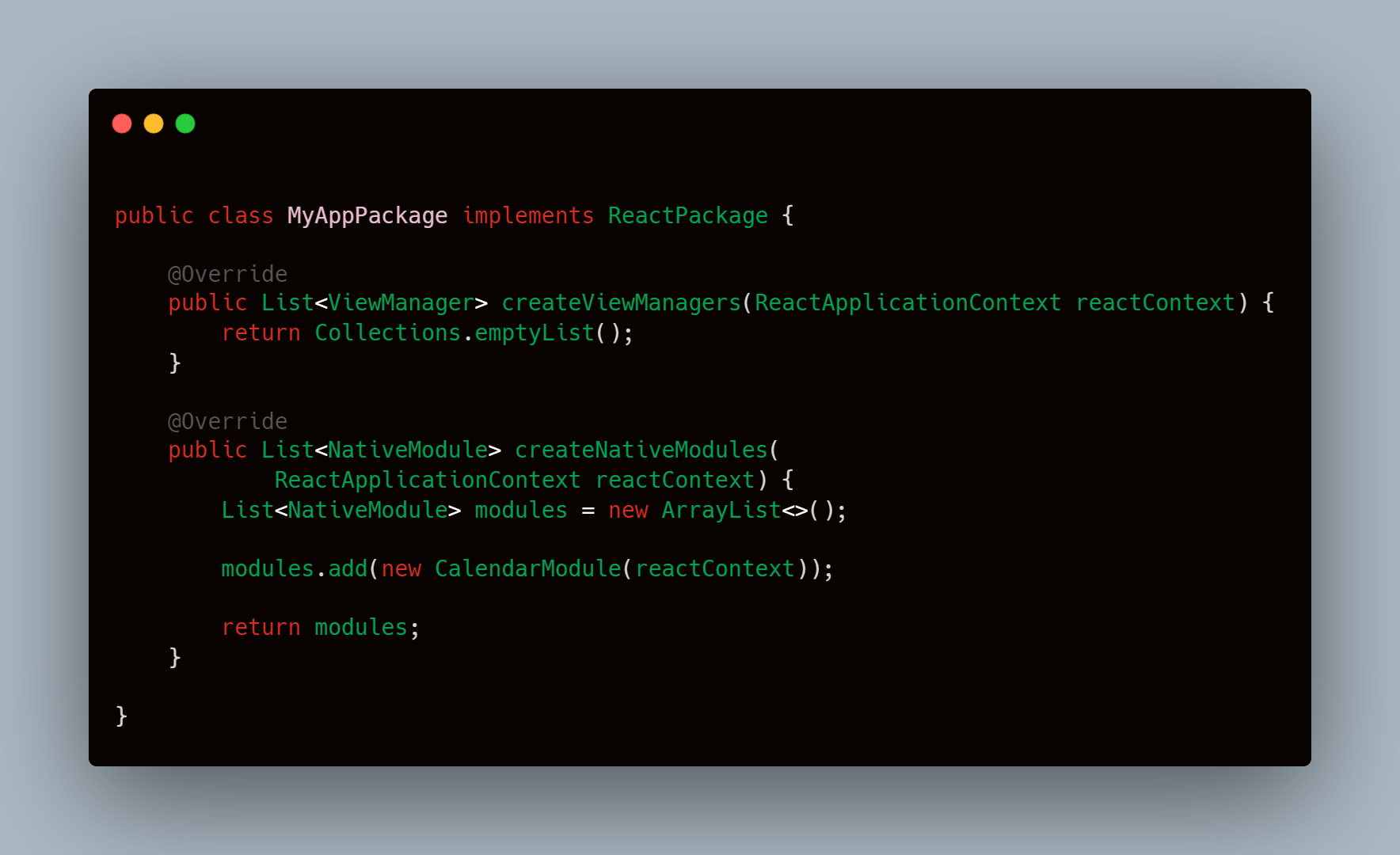
We're done on the Java side. Back to RN now.
To use the new created module - first import NativeModules from react-native and destructure your CalendarModule.
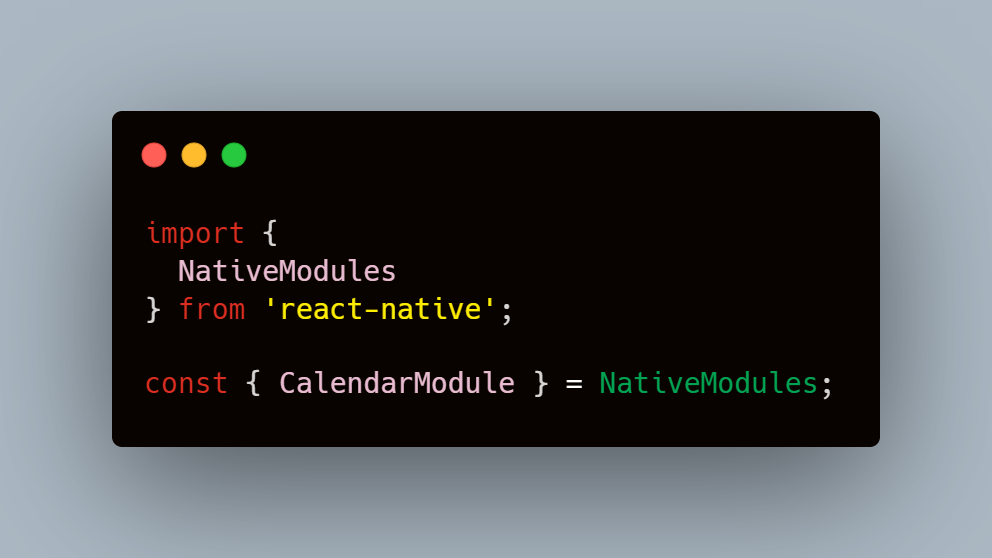
Lastly call the exposed API to create a calendar event.
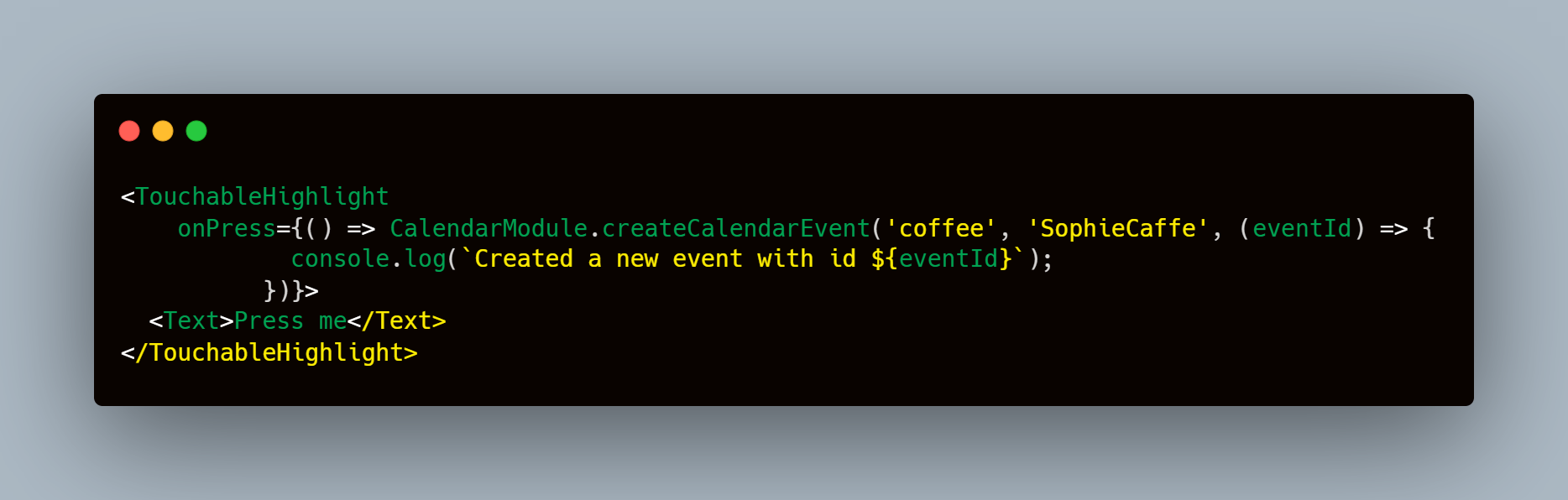
So the method we wrote in Java (createCalendarEvent) is the one we're calling. The rest is just part of the setup.
Here we would also receive a callback for knowing that the event is now stored, so the flow can continue.